BME280 - temperature, humidity and pressure sensor
In this tutorial we are going to meausure temperature, humidity and pressure using the BME280 sensor.
Prerequisites
This tutorial assumes that you have done the i2c tutorial.
We recommend to have read the packages tutorial, since we are going to use a package for the BME280 driver.
Setup
Connect the BME280 to the ESP32 as follows:
- VCC to 3V3
- GND to GND
- SCL to pin 25 (yellow on sparkfun boards)
- SDA to pin 26 (blue on sparkfun boards)
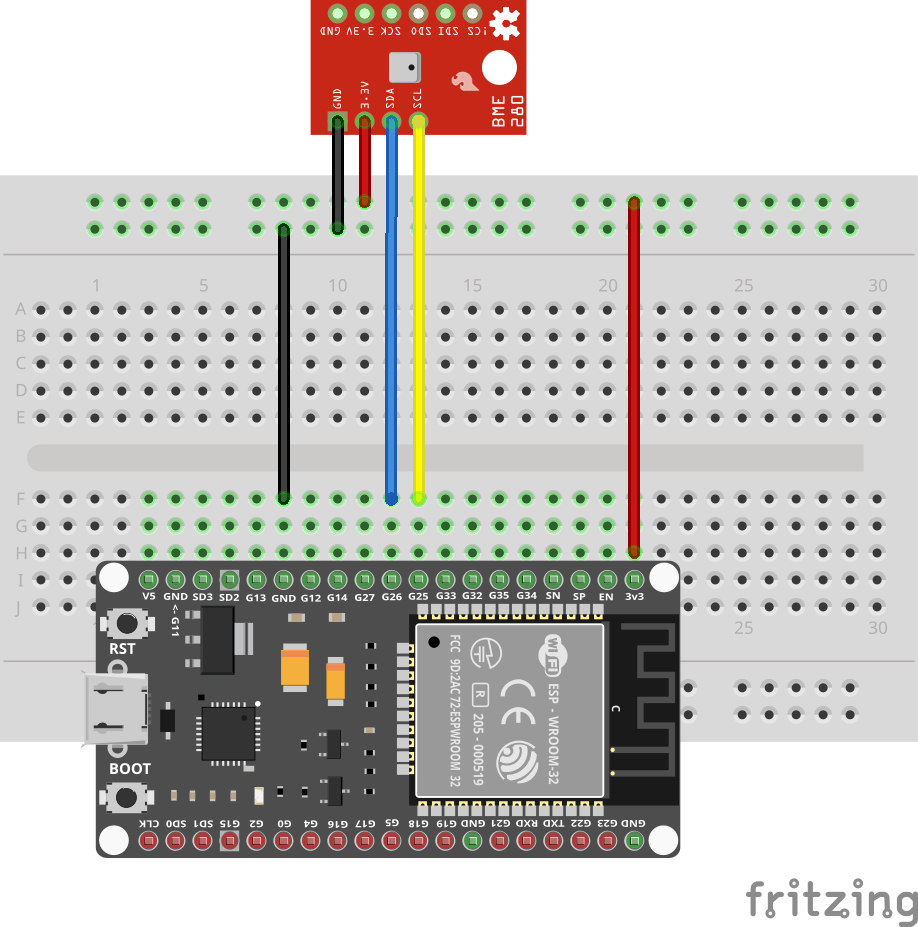
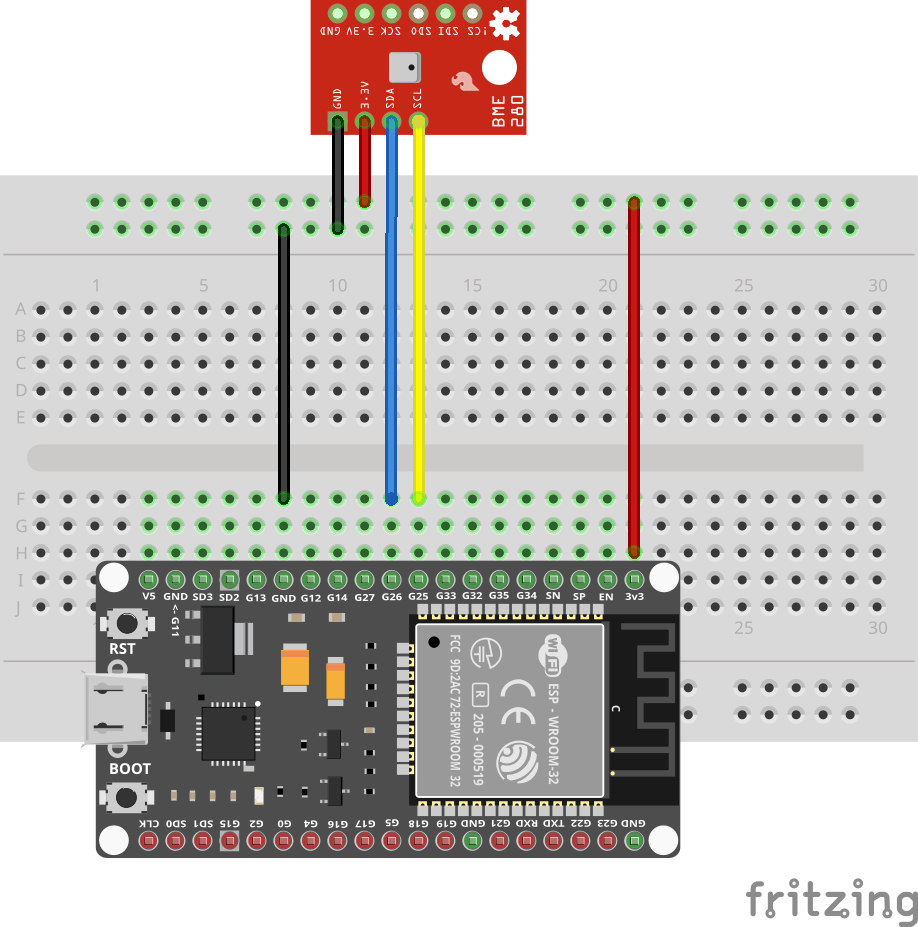
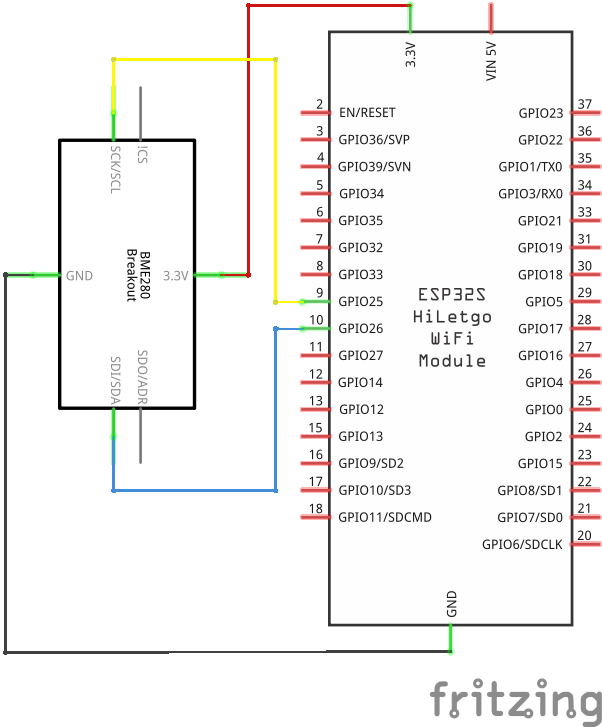
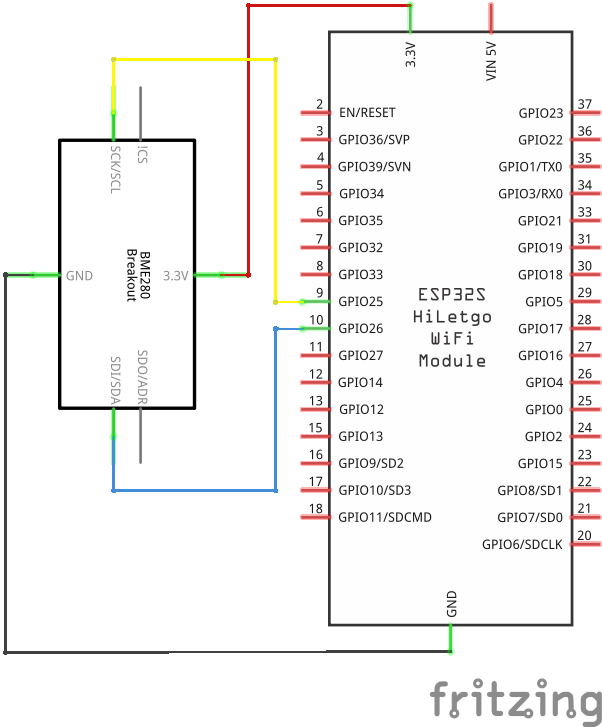
Make sure the device is found by executing the list program from the i2c tutorial. It should print one of the BME280 I2C addresses: 0x76 (118) or 0x77 (119).
Packages
The driver for the BME280 is not part of the standard Toit distribution, but must be downloaded as package. See the packages tutorial for details.
We are using the bme280 package. To install it, run the following command in your project directory:
You can probably just write jag pkg install bme280
, but the full ID together
with the version is more explicit, and will make sure you get the right package.
Code
Create a new file bme280.toit
and start jag watch bme280.toit
to run
the program whenever you save.
import gpio import i2c import bme280 main: bus := i2c.Bus --sda=gpio.Pin 26 --scl=gpio.Pin 25 // Use 'I2C-ADDRESS' if your device has address 0x76 (118). // Use 'I2C-ADDRESS-ALT' if your device has address 0x77 (119). device := bus.device bme280.I2C-ADDRESS driver := bme280.Driver device print "$driver.read-temperature C" print "$driver.read-pressure Pa" print "$driver.read-humidity %" driver.close bus.close
The program first instantiates an I2C bus object, which it uses to get a handle to the device with the BME280 address. It then passes this device to the BME280 driver. At that point the driver configures the sensor.
When read-temperature
, read-pressure
and read-huminity
is called, the driver then requests the corresponding values
from the device. There is some computation to convert the
raw values to human understandable values, but that's hidden
by the driver.
Note that this program uses string interpolation.