HTTP
The ESP32 comes with integrated WiFi and Bluetooth. In this tutorial we are going to take advantage of the WiFi and do an HTTP GET request to get interesting facts.
Prerequisites
We assume that you have set up your development environment as described in the IDE tutorial.
We also assume that you have flashed your device with Jaguar and that you are familiar with running Toit programs on it. If not, have a look at the Hello world tutorial.
Note that you can do this tutorial without a device. In that case,
you need to use the -d host
option whenever you invoke
jag run
. The program will then run on your computer instead of on
a device.
Packages
The HTTP functionality is not part of the core libraries and must be imported as a package. See the packages tutorial for details.
We are using the http package. To install it, run the following command:
We will also use the certificate-roots package:
Code
Start a new Toit program http_get.toit
and watch it with Jaguar.
import http import net import certificate-roots import encoding.json URL ::= "uselessfacts.jsph.pl" PATH ::= "/random.json?language=en" main: certificate-roots.install-common-trusted-roots network := net.open client := http.Client.tls network request := client.get URL PATH decoded := json.decode-stream request.body print decoded["text"]
Doing an HTTP GET is straightforward and consists of calling
get
on an HTTP client object.
However, things are complicated by two facts:
- we need to use the HTTPS connection, and
- the returned data is given as a byte-array and must be decoded.
In order to verify the authenticity of the https server we need
to have the certificate of the authenticity that signed the server's
own certificate. For simplicity we just install the "common trusted roots"
of the certificate-roots
package. This is a set of root certificates
that cover the majority of the internet.
In the case of https://uselessfacts.jsph.pl the root authority is "ISRG Root X1". This information can be extracted by looking at the site's certificate in Chrome:
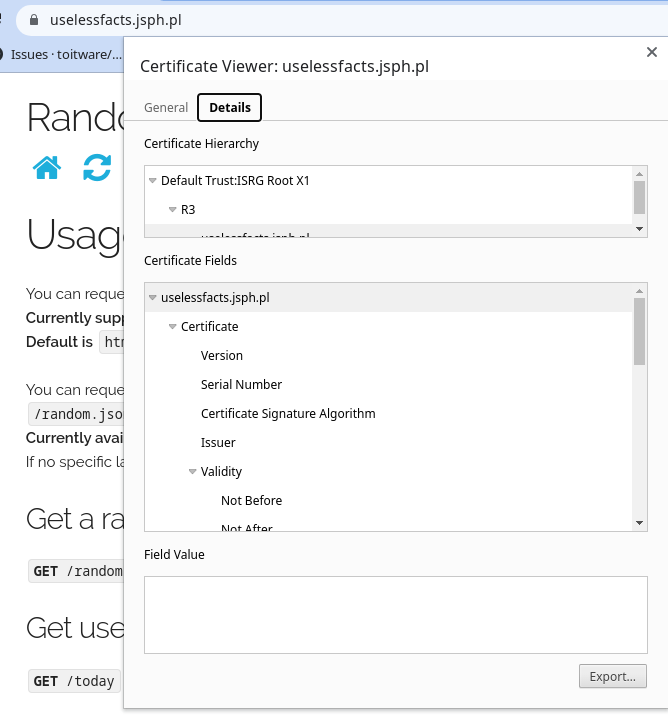
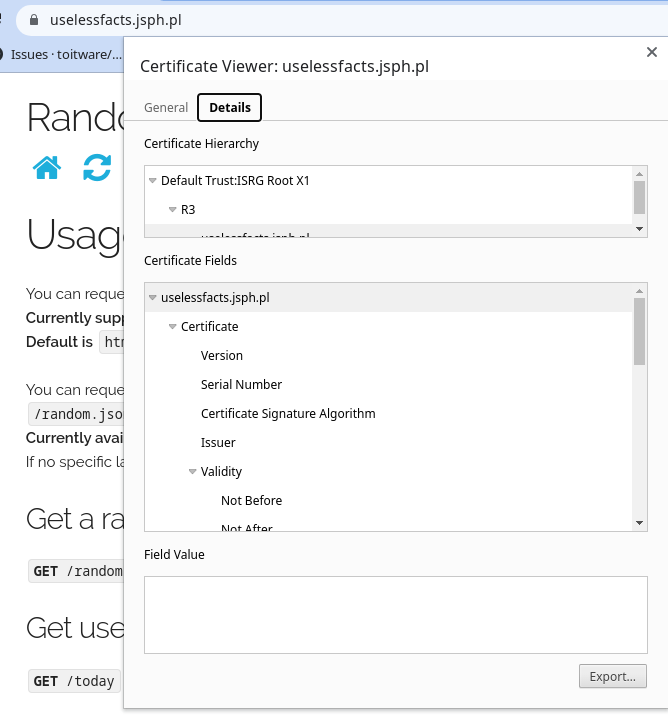
The certificate-roots
package is maintained by Toitware and includes roots
that are shipped with Mozilla's Firefox and should thus be safe to use.
Decoding the given data as JSON is straightforward with the decode-stream
functionality of the json
library (imported with encoding.json
).
The server is supposed to return an array of exactly one string item.
As such the output of this program could look something like:
[jaguar] INFO: starting program 6a63204c-1c98-519d-bdaa-90e52b491fb4 Until 1994, world maps and globes sold in Albania only had Albania on them.
Exercises
It's almost impossible to damage the ESP32 without connecting hardware to it.
- Fetch different interesting data. The repository https://github.com/public-apis-dev/public-apis has lots of public services that could be used for this purpose.
- Show the data on a display.
- Use a button to fetch a new fact every time the button is pressed.