DS18B20
The DS18B20 is a cheap temperature sensor. Similar to the DHT11 it only needs one wire (in addition to VCC and GND) for communication. In fact, the used 1-wire protocol even has a mode where the VCC line isn't needed. In that "parasitic" mode the sensor is powered by the DQ line. However, in this tutorial we are going to power the sensor via VCC (as this is how most sensors are packaged).
The 1-wire protocol is standardized and used by different sensors that are usually cheap and don't need much power. See, for example, Maxim's 1-wire products.
Prerequisites
We assume that you have set up your development environment as described in the IDE tutorial.
We also assume that you have flashed your device with Jaguar and that you are familiar with running Toit programs on it. If not, have a look at the Hello world tutorial.
Setup
Connect the DS18B20 sensor as follows:
- Connect the sensor's VCC/+ pin to the ESP32's 3.3V pin.
- Connect the sensor's GND/- pin to the ESP32's GND pin.
- Connect the sensor's DATA/OUT/S pin to pin 32.
There are many different variants of the DS18B20 modules and they don't always have the same pin-out. Be sure to check the pin-out of your module before connecting it.
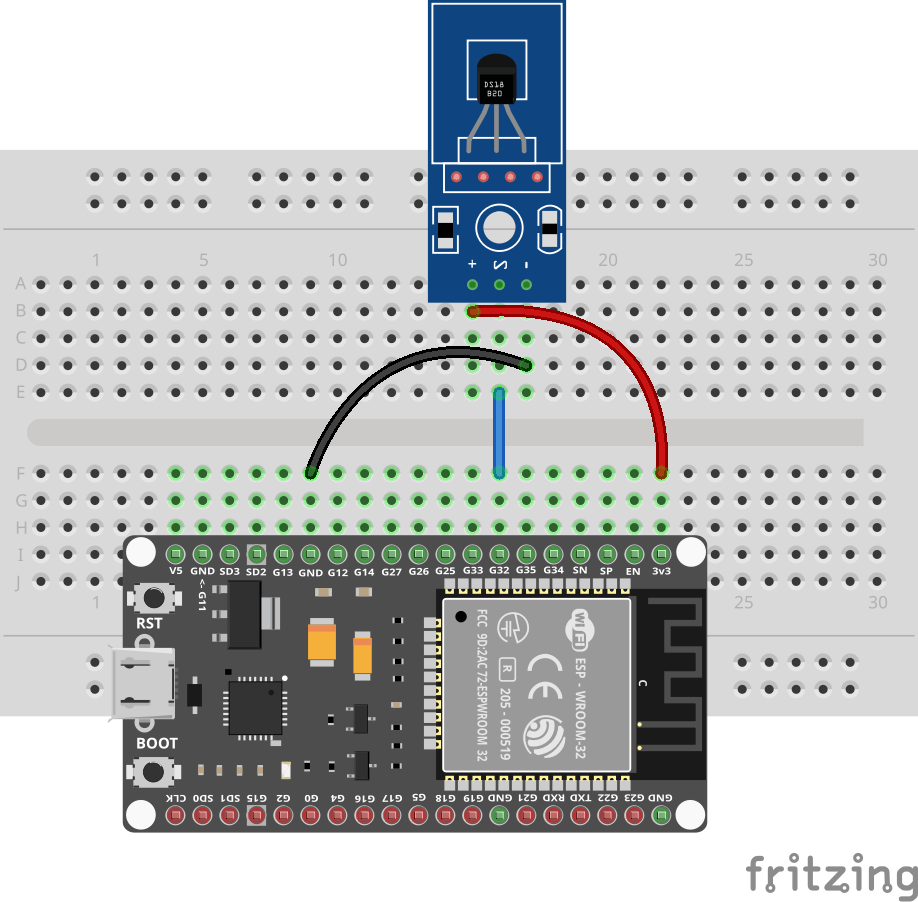
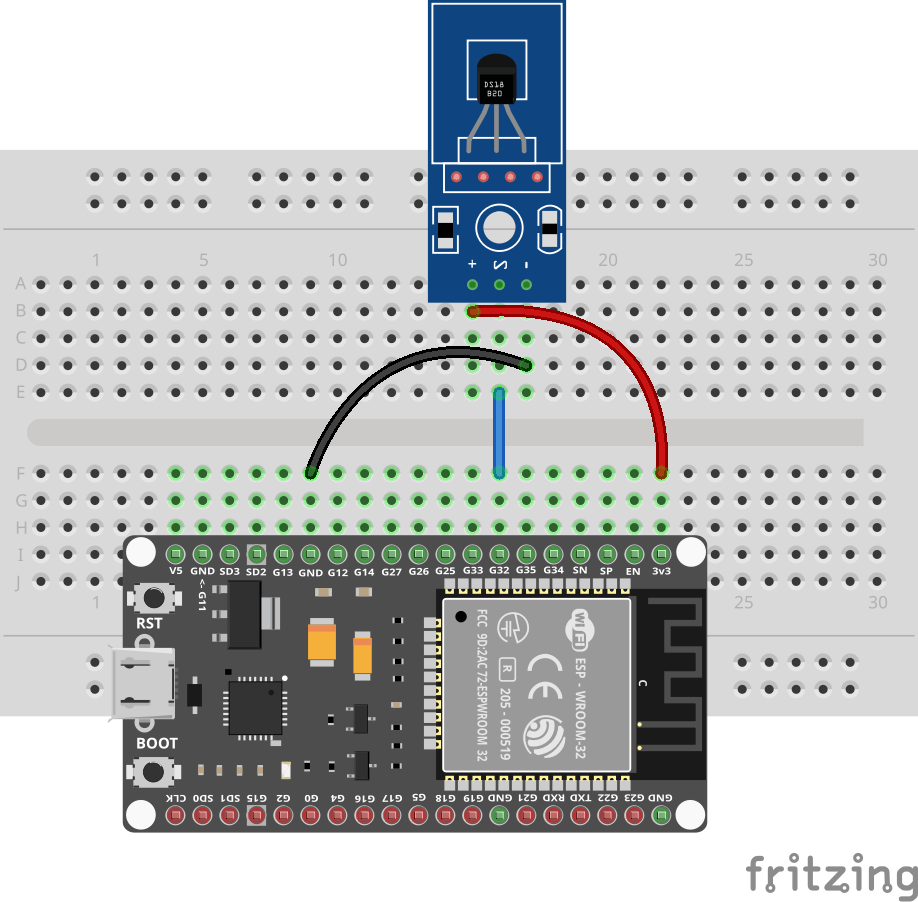
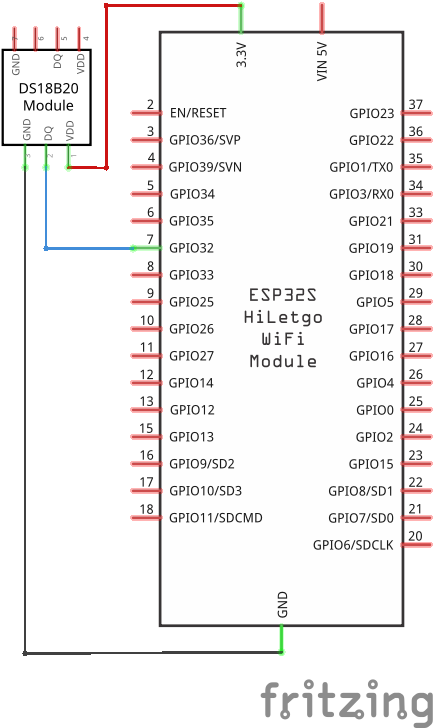
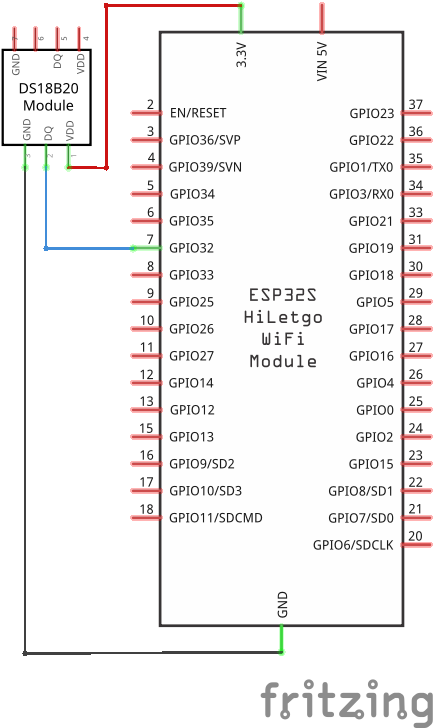
Packages
The driver for the DS18B20 is not part of the standard Toit distribution, but must be downloaded as package. See the packages tutorial for details.
We are using the ds18b20 package. To install it, run the following command in your project directory:
You can probably just write jag pkg install ds18b20
, but the full ID together
with the version is more explicit, and will make sure you get the right package.
Code
Write the following ds18b20.toit
file and watch it with Jaguar.
import ds18b20 show Ds18b20 import gpio GPIO-PIN-NUM ::= 32 main: pin := gpio.Pin GPIO-PIN-NUM ds18b20 := Ds18b20 pin (Duration --ms=500).periodic: print "Temperature: $(%.2f ds18b20.read-temperature) C"
The code allocates a ds18b20 object for the given pin and then reads the temperature every 500ms.
Exercises
As long as the connections were done correctly you can't damage your hardware by changing your program.
- Connect an additional LED (don't forget the resistor) and turn it on when the temperature reaches a certain threshold.
- Show the current temperature on a display.